6.4 - Creating a scrollable side panel
Here you will learn how to make a scrollable side panel like the one in my mod menu.
First we need to create the main panel:
// creating the main panel
var sidePanel = RegisterNewPanel("SidePanel", true) // registering the panel and enabling input listening
.Pivot(0, 1) // setting the right pivot for top left corner of the screen (refer to Panels lesson if you don't udnerstand)
.Anchor(AnchorType.TopLeft)
.Background(Color.red, EBackground.None) // changing panel color to red and styling it with straight borders
.Size(500) // specifying the width of the panel (the height can be omitted)
.Vertical(5, "EC") // specifying that the panel must have a vertical layout (so it's content is distributed vertically)
.VFill(); // specifying that the panel must fill the screen vertically
Then we create an element which I don't really know what is but we will call it the scrollbar and add it to the main panel:
// creating the scrollbar?
var scrollBar = SDiv.FlexHeight(1);
sidePanel.Add(scrollBar);
Then we can create a scrollable container which will be inside the main panel we created before:
// creating the container which will be inside the main panel
var settingsScroll = SScrollContainer // declaring the container as Scrollable
.Dock(EDockType.Fill) // specifying that the container will fill all of the available space
.Background(Color.green, EBackground.None) // styling the container
.Size(-20, -20) // adding some padding from the main container
.As();
Lastly we can further personalize the container and add it to the "scrollbar" element:
// further personalizing the panel to make it look better
settingsScroll.ContainerObject.Spacing(80); // the space between each vertical ui element
settingsScroll.ContainerObject.PaddingHorizontal(10); // the space on left and right from the scrollable container towards the ui elements
settingsScroll.ContainerObject.PaddingVertical(40); // the space on top and bottom from the scrollable container towards the ui elements
scrollBar.Add(settingsScroll); // adding the container to the scrollbar element
If we then add ui elements like toggles to the settingsScroll
scrollable container you will see that we will be able to scroll down:
// adding ui elements to the scrollable container
for (int i = 0; i < 15; i++)
{
settingsScroll.Add(SToggle.Text("Toggle"));
}
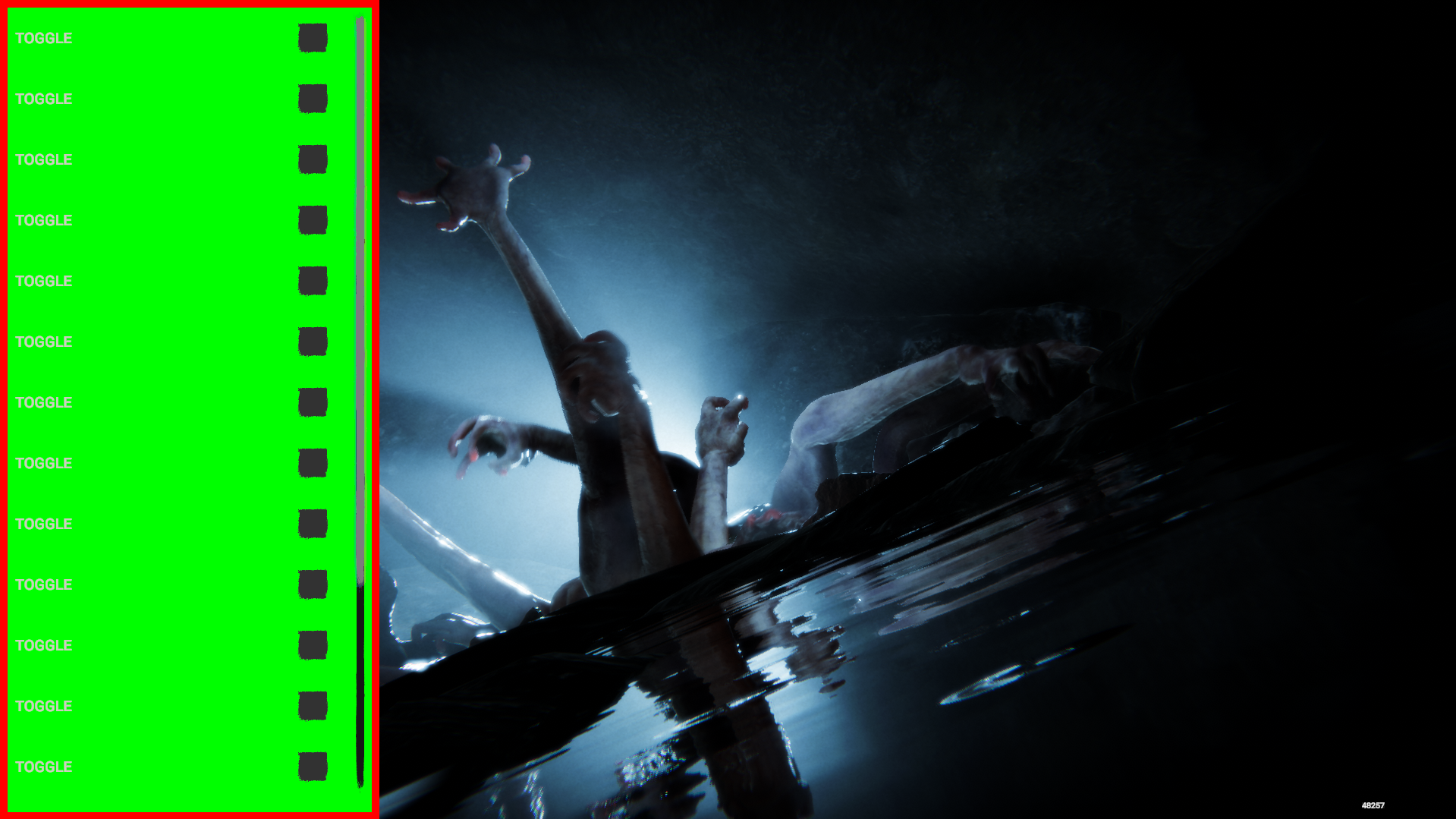
Complete code below:
// creating the main panel
var sidePanel = RegisterNewPanel("SidePanel", true) // registering the panel and enabling input listening
.Pivot(0, 1) // setting the right pivot for top left corner of the screen (refer to Panels lesson if you don't udnerstand)
.Anchor(AnchorType.TopLeft)
.Background(Color.red, EBackground.None) // changin panel color to red and styling it with straight borders
.Size(500) // specifying the width of the panel (the height can be omitted)
.Vertical(5, "EC") // specifying that the panel must have a vertical layout (so it's content is distributed vertically)
.VFill(); // specifying that the panel must fill the screen vertically
// creating the scrollbar?
var scrollBar = SDiv.FlexHeight(1);
sidePanel.Add(scrollBar);
// creating the container which will be inside the main panel
var settingsScroll = SScrollContainer // declaring the container as Scrollable
.Dock(EDockType.Fill) // specifying that the container will fill all of the available space inside the main panel
.Background(Color.green, EBackground.None) // styling the container
.Size(-20, -20) // adding some padding from the main container
.As();
// further personalizing the panel to make it look better
settingsScroll.ContainerObject.Spacing(80); // the space between each vertical ui element
settingsScroll.ContainerObject.PaddingHorizontal(10); // the space on left and right of the ui elements from the scrollable container
settingsScroll.ContainerObject.PaddingVertical(40); // the space on top and bottom of the ui elements from the scrollable container
scrollBar.Add(settingsScroll); // adding the container to the scrollbar
// adding ui elements to the scrollable container
for (int i = 0; i < 15; i++)
{
settingsScroll.Add(SToggle.Text("Toggle"));
}
The same principles should apply for a centered panel etc.